Login
To successfully authenticate, a user must have a valid Firstock Trading Account and an active subscription to Firstock API Services. Login APIs facilitate the authentication process.
Key generation
For the Login process we require to generate the appkey and vendor code by logging in with the firstock credentials in the give link Key Generation.
Step 1: Go to the connect key generation and enter the firstock credentials
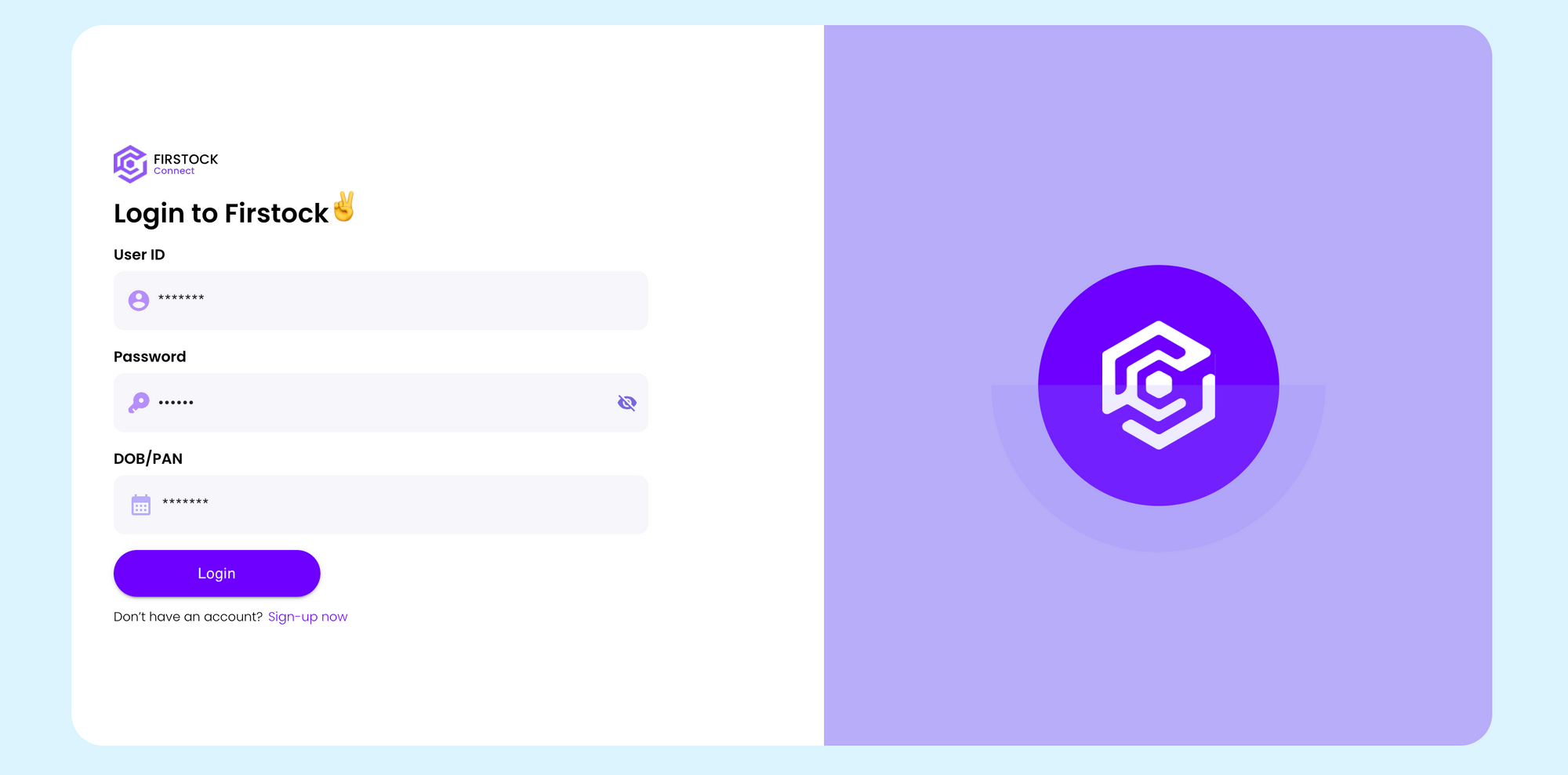
Step 2: Once logged in you will find the appkey and vendor code, please copy it and paste it into the fields in the login API.
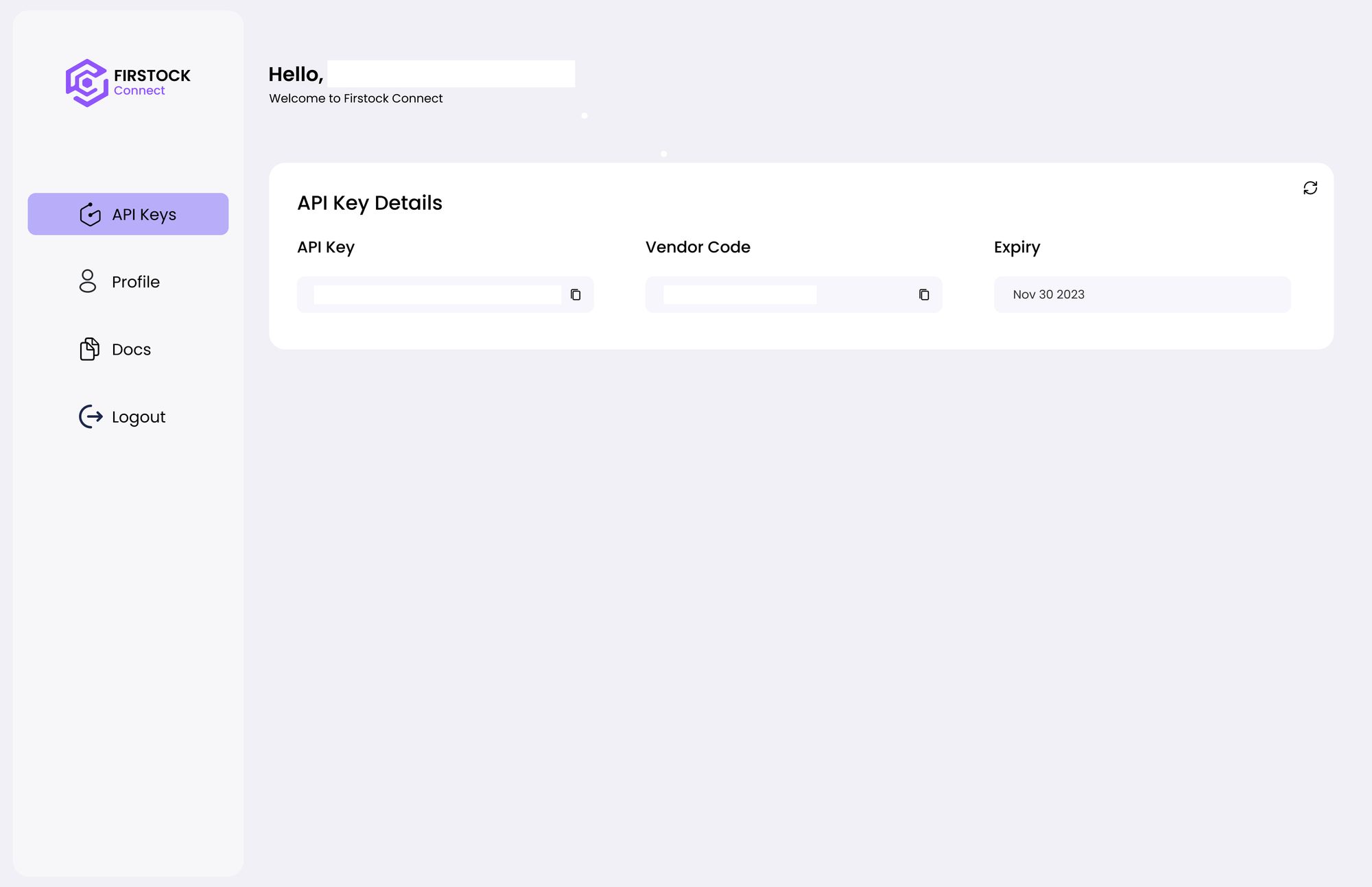
Upon a successful login, a status of success is returned along with a data object containing user-specific parameters.
In the new V4 the password should be converted to SHA256 before passing to the login API in the cURL request, as for the packages the password will be internally converted and hit to the login request
Note: The new python code allows multiuser login functionality, where the user can login to multiple account simultaneously, but in the current version the user need to input userId in the rest of the code.
Password encryption
Please find the link below for password conversion
Method: POST
https://connect.thefirstock.com/api/V4/login
Request details
Parameter | Description | Example |
---|---|---|
userId string |
Identifier for the user upon login, unique to each user in the system. |
AA0013 |
password string |
User generated secret | trade@45 |
TOTP string |
Dynamic, time-sensitive passcode used for secure authentication. |
897623 |
apiKey string |
Unique identification key from the firstock | 9966df4479ddaf5ab5 |
vendorCode string |
Unique user code obtained in key generator | AA0013_API |
Libraries and SDKs
from thefirstock import thefirstock
login = thefirstock.firstock_login(
userId="{{userID}}",
password="{{Password}}",
TOTP="{{TOTP}}",
vendorCode="{{vendorCode}}",
apiKey="{{apiKey}}",
)
curl --location --request POST 'https://connect.thefirstock.com/api/V4/login' \
--header 'Content-Type: application/json' \
--data-raw '{
"userId": "{{userID}}",
"password": "{{Password}}", #Convert to SHA256
"TOTP": "{{TOTP}}",
"vendorCode": "{{vendorCode}}",
"apiKey": "{{apiKey}}"
}'
const Firstock = require("thefirstock");
const firstock = new Firstock();
firstock.login(
{
userId: "AA123",
password: "f4504e233f48d27328d5e53766d9141c50e8f43aec249537c3a4d9e94ccfdbef",
TOTP: "1234698",
vendorCode: "AA123_API",
apiKey: "NVDewefds2343q2334",
},
(err, result) => {
console.log("Error, ", err);
console.log("Result: ", result);
}
);
using thefirstock;
class Program
{
public static void Main()
{
Firstock firstock = new Firstock();
var result = firstock.login(
userId: "AA123",
password: "f4504e233f48d27328d5e53766d9141c50e8f43aec249537c3a4d9e94ccfdbef",
TOTP: "1234698",
vendorCode: "AA123_API",
apiKey: "NVDewefds2343q2334"
);
}
}
Success response details
Parameter | Description | Example |
---|---|---|
status string |
Provides the result of the API | success |
data.actid string |
actid is the identity given during registration with Firstock |
AA0013 |
data.userName string |
Name of the user | Raj Kiran |
data.susertoken string |
It used for authenticate and access other API and is also called jKey |
9966df4479ddaf5ab5 |
data.email string |
Email Id of the user | [email protected] |
{
"status": "success",
"data": {
"actid": "AA0013",
"userName": "AA Kiran",
"susertoken":"dc6b91b7d4059be1d875e83774c5bb3374",
"email": "[email protected]"
}
}
Failure response details
Parameter | Description | Example |
---|---|---|
status String |
Provides the status of the API | failed |
code String |
Povides the HTTP code of the API | 401 |
name String |
Provides the type of error | INVALID_PASSWORD |
error.field String |
Tell in which the error was caused | password |
error.message String |
Provides the reason for the error | password parameter is invalid |
{
"status": "failed",
"code": "401",
"name": "INVALID_PASSWORD",
"error": {
"field": "password",
"message": "password parameter is invalid"
}
}